Handle Dependencies
The project uses CocoaPods to manage its dependencies:
WHAT IS COCOAPODS
CocoaPods is a dependency manager for Swift and Objective-C Cocoa projects. It has over 72 thousand libraries and is used in over 3 million apps. CocoaPods can help you scale your projects elegantly.
The CocoaPods file is Podfile
, which describes the iOS version (7.0)
to use, and the dependencies name, version, and source:
1 | platform :ios, '7.0' |
The first step to build it is installing the dependencies. After installing CocoaPods, I ran pod install
and got some errors:
1 | $ pod install |
There are some bugs to fix 😃
Bugfix
1 | [!] Could not automatically select an Xcode workspace. Specify one in your Podfile like so: |
In this project, the .xcworkspace
file is kdeconnect-ios.xcworkspace
. So, I add workspace 'kdeconnect-ios'
into the file.
1 | [!] The platform of the target `Pods` (iOS 7.0) may not be compatible with `InAppSettingsKit (2.15)` which has a minimum requirement of iOS 8.0. |
The target OS version should be updated. I directly update it to platform :ios, '12.0'
.
1 | [!] The abstract target Pods is not inherited by a concrete target, so the following dependencies won't make it into any targets in your project: |
This message indicates that the pods
(dependencies) do not have a target; according to the CocoaPod doc, I should add target "kdeconnect-ios"
and wrap all the pods with a do ... end
body.
Final Podfile
As a result, the Podfile
should be:
1 | workspace 'kdeconnect-ios' |
After fixing, the output seems normal:
1 | $ pod install |
Build with XCode
Without error in installing dependencies, it is possible to open and build it with XCode (though it could be the worst IDE in the world).
Identifier
The first issue occurred while I click Build
:
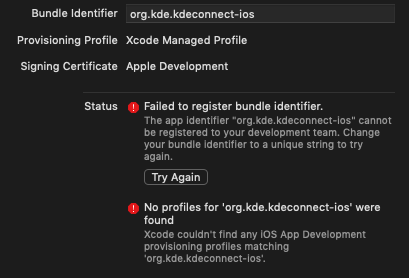
1 | Failed to register bundle identifier. |
Apple wants to generate a self-signed certificate for my Apple account and the application. But it cannot handle the identifier. I change it to org.kde.kdeconnect.ios
and click Try again
. It works.
Multiple commands produce
Then, rebuild. And another one came out:
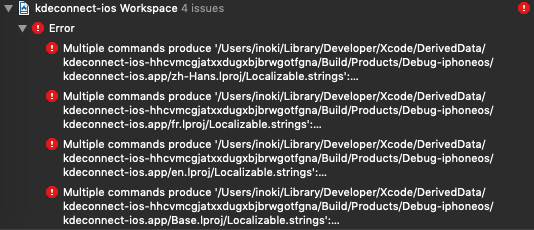
1 | Multiple commands produce '/Users/inoki/Library/Developer/Xcode/DerivedData/kdeconnect-ios-hhcvmcgjatxxdugxbjbrwgotfgna/Build/Products/Debug-iphoneos/kdeconnect-ios.app/zh-Hans.lproj/Localizable.strings': |
To fix it, I refer this GitHub issue and change the build system to the legacy one:
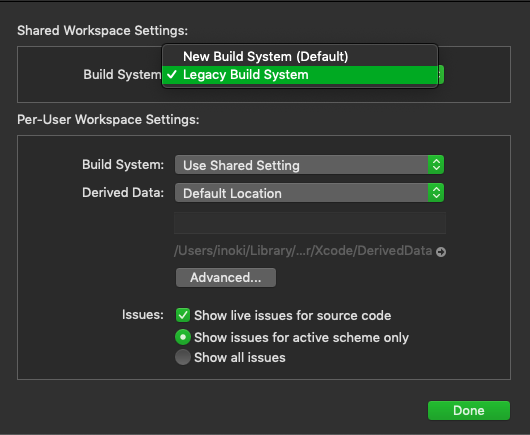
Missing header
After that, another error occurs:

1 | kdeconnect-ios/AppSettingViewController.m:27:9: 'IASKPSTitleValueSpecifierViewCell.h' file not found |
This may be an update of one dependency; remove this import line:
1 | #import "IASKPSTitleValueSpecifierViewCell.h" |
Link error, disable Bitcode
I rebuilt it, but another one came…
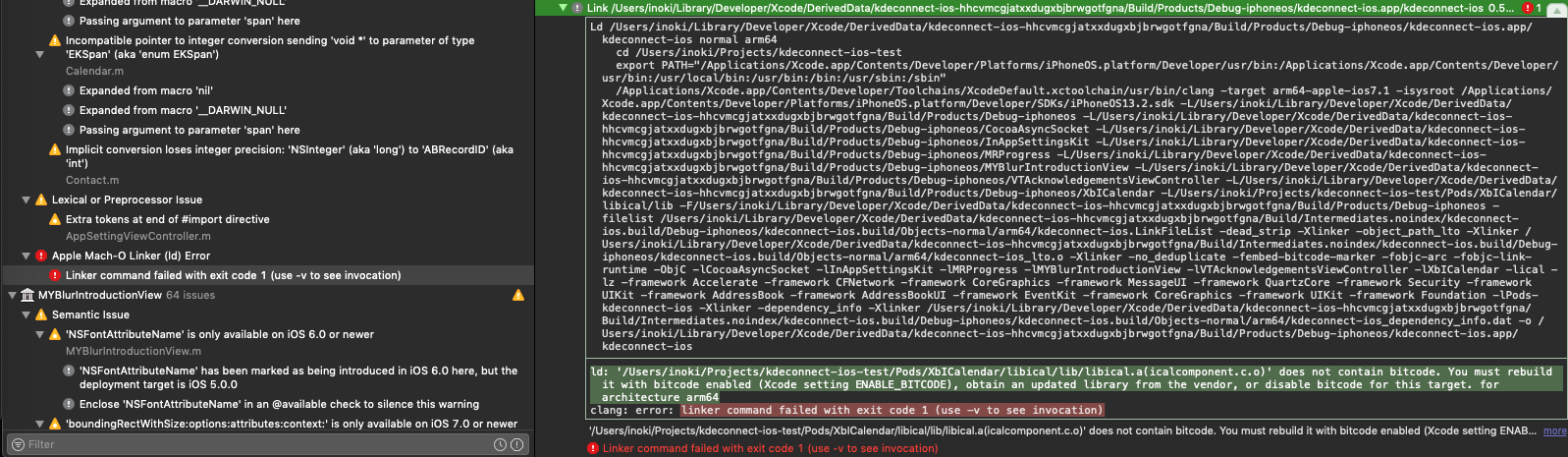
1 | ld: '/Users/inoki/Projects/kdeconnect-ios-test/Pods/XbICalendar/libical/lib/libical.a(icalcomponent.c.o)' does not contain bitcode. You must rebuild it with bitcode enabled (Xcode setting ENABLE_BITCODE), obtain an updated library from the vendor, or disable bitcode for this target. for architecture arm64 |
Bitcode may be a feature, but I want to get it to build as soon as possible. So, I disable it in Build Settings->Build Options
:
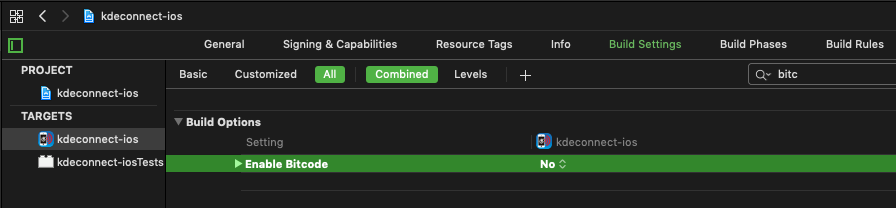
Link with WebKit
Another link error occurs. It is about the WebView stuff:
1 | Undefined symbols for architecture arm64: "_OBJC_CLASS_$_WKWebView" |
It seems that the WebKit framework is not included in the project. So, add it in Build Phases->Link Binary with Libraries
:
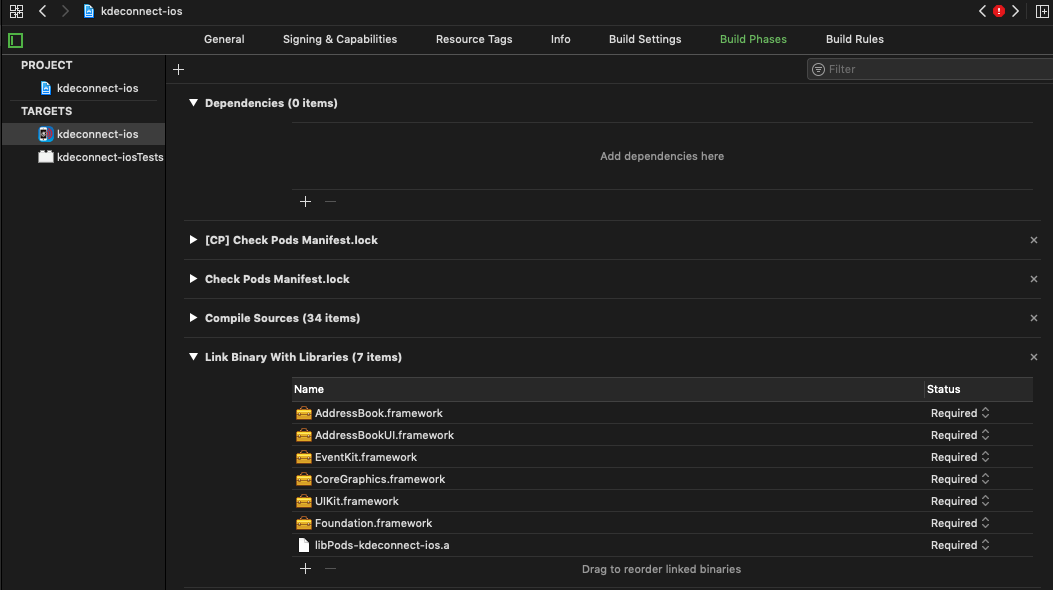
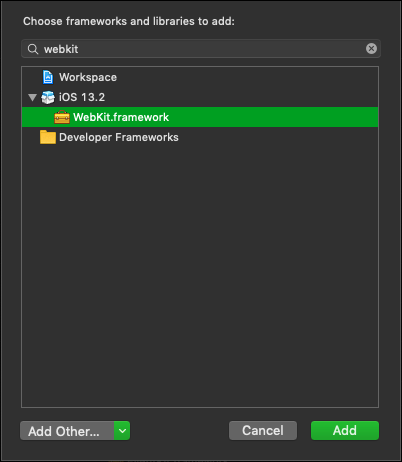
File(Pods-acknowledgements.plist, Pods-resources.sh) not found
Finally, at the end of building phases, the error came out:
1 | Pods/Pods-acknowledgements.plist:0: Reading data: The file “Pods-acknowledgements.plist” couldn’t be opened because there is no such file. |
This could be an error caused by CocoaPod version. To fix it, copy the files in Pods/Target Support Files/Pods-kdeconnect-ios
to Pods/
:
1 | cp Pods-kdeconnect-ios-acknowledgements.plist ../../Pods-acknowledgements.plist |
Finally, it works 😃